Let’s look into the Fundamental concepts of React Js for beginners by Lia Infraservices – The top Mobile App Development Company in Chennai. React is a front-end open source JavaScript library for creating user interfaces (UI) components. Complex user interfaces are broken down into small “components,” which are separate pieces of “reusable codes”.
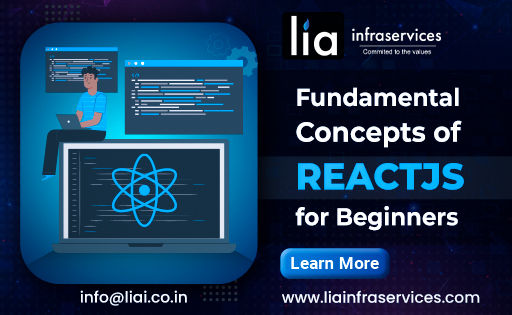
The components are self-contained and reusable. They can be “JavaScript functions” or “JavaScript classes”.
Class components provide certain key functions that function components lack, whereas function components are mostly simple JavaScript.
/React is designed around Reusable concepts
Getting started with React Js:
Open terminal in visual studio code :
Install create-react-app by running this command in your terminal:
C:\Users\LIA>npm install -g create-react-app
Run this command to create a React application :
C:\Users\LIA>npx create-react-app myfirstreact
Run this command to execute the React application:
C:\Users\LIA>npm start
Hello world Example:
Change the code of App.js from src folder
import React, { Component } from ‘react’;
//import ‘./App.css’;
class App extends Component {
render() {
return (
<div className=”App”>
<h1>Hello World!</h1>
</div>
);
}
}
export default App;
Output:
If you did not “export default App”
export default App;
It returns an error.
2. Folder structure:
What is await in react?
The await operator is used to wait for a Promise. It can only be used inside an async function (The keyword async is used to make a function asynchronous).The await keyword will ask the execution to wait until the defined task gets executed.
Javascript Hoisting:
Hoisting is a default behaviour of moving all the declarations at the top of the scope before code execution.
Ex:
function catName(name) {
console.log(“My cat’s name is ” + name);
}
catName(“Tiger”);
Or
catName(“Tiger”);
function catName(name) {
console.log(“My cat’s name is ” + name);
}
Call function using before or after declaration Does not return any error, because of hoisting
But,but call before function Expression returns error ex:
catName(“Tiger”); // Returns undefined, as only declaration was hoisted, no initialization has happened at this stage
Let catName = function(name) {
console.log(“My cat’s name is ” + name);
}
Var,let and const variables:
var declarations are globally scoped or function scoped while let and const are block scoped. var variables can be updated and re-declared within its scope; let variables can be updated but not re-declared; const variables can neither be updated nor re-declared. They are all hoisted to the top of their scope.
3. Component:
A Component is one of the core building blocks of React.
In other words, we can say that every application you will develop in React will be made up of pieces called components.
Components make the task of building UIs much easier.
You can see a UI broken down into multiple individual pieces called components and work on them independently and merge them all in a parent component which will be your final UI.
A) Functional Components:
Functional components are simply javascript functions. We can create a functional component in React by writing a javascript function. These functions may or may not receive data as parameters
Example:
B) Class Components:
The class components are a little more complex than the functional components. The functional components are not aware of the other components in your program whereas the class components can work with each other. We can pass data from one class component to other class components
Ex:
The component’s name must start with an upper case letter
The component has to include the extends React.Component statement, this statement creates an inheritance to React.Component,
The component also requires a render() method, this method returns HTML.
When you use a constructor function, it should contain super() which executes the parent component’s constructor function, and your component has access to all the functions of the parent component (React.Component).
Probs:
Short form of Properties
Readonly
Pass parameters to functions
Ex:
Class based probs:
App.js
Index.js
(need to use “this” before using probs)
Function based probs:
App.js
Index.js
(pass probs in function)
4. State:
State(local container) is a JavaScript object that stores a component’s dynamic data and determines the component’s behaviour. Because state is dynamic, it enables a component to keep track of changing information in between renders and for it to be dynamic and interactive. State can only be used within a class component.
Ex:
Output:
5) Rendering a component:
Ex: code in index.js file
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
const element = <Welcome name=”Sara” />;
ReactDOM.render(element, document.getElementById(‘root’));
Save the css with proper syntax:
Execute the command npm run watch and save the “less file” , css file will be generated automatically and need to import the css file in app.js to use css
5.a) Component Lifecycle:
Each class component has separate life cycle
Phases:
Mount (Component created and inserted in DOM)
Update (Undergoes growth by being updated via changes in probs and state)
Unmount(where component is removed fro DOM)
5.b) Order of Execution:
Case 1: Use Parent component
Constructor() -> render() -> componentDidMount()
Case 2: use child component
Constructor() -> Parent Render() -> Child component render -> Parent componentDidMount()
Case 3: Use child componentDidMount
Constructor() -> Parent Render() -> Child component render ->Child componentDidMount() -> Parent componentDidMount() ( ->Child component render (This stage occur only when child component contain an continuous execution function like timer))
Case 4: use componentWillUnmount()
Constructor() -> Parent Render() -> Child component render ->Child componentDidMount() -> Parent componentDidMount() ->componentWillUnmount()
componentWillMount():
It executes before render(), This method can not used for current version
componentDidUpdate():
Handle the operations when user interact (state and probs change)
componentDidMount():
Server side communication can be effectively handled in this phase (call defaultly after constructor execution)
Conclusion:
Although this article does not cover everything that you need to know about React Js. The Fundamental concepts of React JS for beginners by Lia Infraservices – Leading Mobile App Development Company in Saudi Arabia provides a good starting point for diving into the React JS code.
Don’t forget to check the official React JS for more in-depth information.
Comments